Getting Started
Installing Python
Go to www.python.org and download the latest version of Python (version 3.5 as of this writing). It should be painless to install. If you have a Mac or Linux, you may already have Python on your computer, though it may be an older version. If it is version 2.7 or earlier, then you should install the latest version, as many of the programs in this book will not work correctly on older versions.
IDLE
IDLE is a simple integrated development environment (IDE) that comes with Python. It’s a program that allows you to type in your programs and run them. There are other IDEs for Python, but
for now I would suggest sticking with IDLE as it is simple to use. You can find IDLE in the Python 3.4 folder on your computer.
When you first start IDLE, it starts up in the shell, which is an interactive window where you can type in Python code and see the output in the same window. I often use the shell in place of my calculator or to try out small pieces of code. But most of the time you will want to open up a new window and type the program in there.
Note At least on Windows, if you click on a Python file on your desktop, your system will run the program, but not show the code, which is probably not what you want. Instead, if you right-click
on the file, there should be an option called Edit with Idle. To edit an existing Python file, either do that or start up IDLE and open the fle through the File menu.
Keyboard shortcuts The following keystrokes work in IDLE and can really speed up your work.
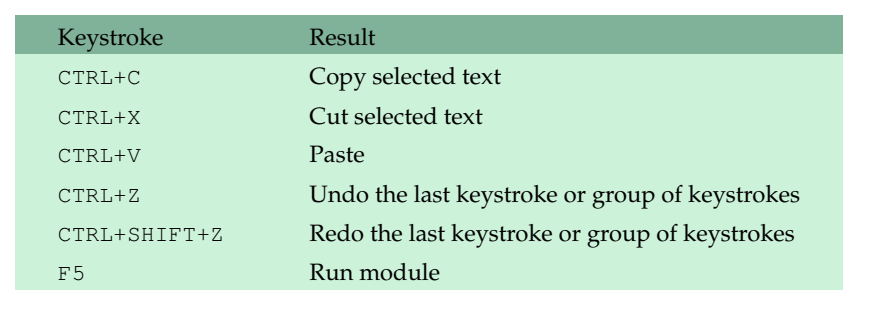
A first program
Start IDLE and open up a new window (choose New Window under the File Menu). Type in the following program.
temp = eval(input('Enter a temperature in Celsius: '))
print('In Fahrenheit, that is', 9/5*temp+32)
Then, under the Run menu, choose Run Module (or press F5). IDLE will ask you to save the fle, and you should do so. Be sure to append .py to the flename as IDLE will not automatically append it. This will tell IDLE to use colors to make your program easier to read.
Once you’ve saved the program, it will run in the shell window. The program will ask you for a temperature. Type in 20 and press enter. The program’s output looks something like this:
Enter a temperature in Celsius: 20
In Fahrenheit, that is 68.0
Let’s examine how the program does what it does. The frst line asks the user to enter a temperature. The input function’s job is to ask the user to type something in and to capture what the user types. The part in quotes is the prompt that the user sees. It is called a string and it will appear to the program’s user exactly as it appears in the code itself. The eval function is something we use here, but it won’t be clear exactly why until later. So for now, just remember that we use it when we’re getting numerical input.
We need to give a name to the value that the user enters so that the program can remember it and use it in the second line. The name we use is temp and we use the equals sign to assign the user’s value to temp.